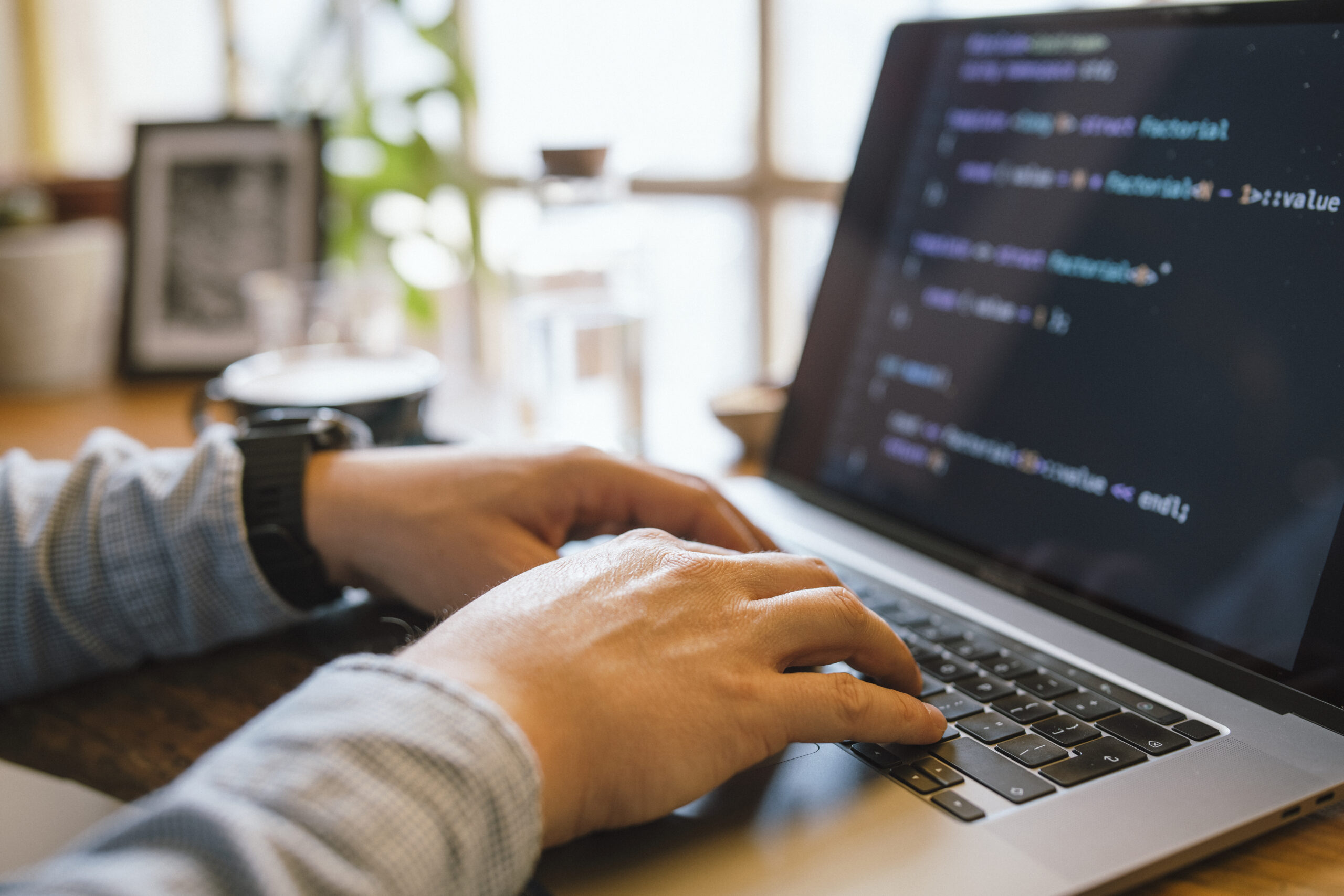
Debugging is Just about the most necessary — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It's not just about repairing damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Consider methodically to unravel complications efficiently. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging techniques can help save several hours of annoyance and radically help your efficiency. Here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Instruments
One of several quickest methods builders can elevate their debugging competencies is by mastering the instruments they use every day. Though producing code is one particular Portion of improvement, knowing the best way to interact with it correctly through execution is equally essential. Modern advancement environments come Geared up with highly effective debugging capabilities — but many builders only scratch the floor of what these instruments can do.
Consider, as an example, an Integrated Enhancement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications help you set breakpoints, inspect the worth of variables at runtime, phase via code line by line, as well as modify code over the fly. When utilised appropriately, they Permit you to observe just how your code behaves in the course of execution, which is a must have for tracking down elusive bugs.
Browser developer equipment, like Chrome DevTools, are indispensable for entrance-conclusion developers. They enable you to inspect the DOM, keep track of community requests, perspective actual-time performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Regulate over jogging procedures and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Edition Management devices like Git to comprehend code historical past, come across the precise instant bugs were introduced, and isolate problematic adjustments.
Finally, mastering your applications means going past default settings and shortcuts — it’s about building an personal expertise in your development environment to ensure that when concerns come up, you’re not misplaced at the hours of darkness. The greater you are aware of your applications, the greater time you may expend resolving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more significant — and often overlooked — ways in helpful debugging is reproducing the condition. In advance of leaping to the code or building guesses, developers require to create a dependable natural environment or circumstance exactly where the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often bringing about squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The greater depth you have, the much easier it turns into to isolate the precise problems under which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your neighborhood surroundings. This could necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions associated. These exams not simply assist expose the challenge but also avoid regressions Sooner or later.
Sometimes, The difficulty may be setting-unique — it might take place only on selected functioning devices, browsers, or less than specific configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It requires patience, observation, as well as a methodical solution. But once you can regularly recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You should use your debugging resources a lot more properly, examination likely fixes safely and securely, and converse additional Plainly with the workforce or people. It turns an summary grievance into a concrete problem — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most respected clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders need to find out to deal with error messages as immediate communications with the technique. They typically let you know exactly what transpired, where it transpired, and often even why it occurred — if you know the way to interpret them.
Start out by looking through the message cautiously As well as in whole. Several builders, particularly when below time tension, look at the very first line and instantly get started generating assumptions. But deeper during the error stack or logs may lie the genuine root trigger. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them very first.
Crack the error down into sections. Can it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate induced it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Finding out to acknowledge these can dramatically hasten your debugging process.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context in which the error happened. Check connected log entries, enter values, and up to date changes within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede more substantial challenges and supply hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, serving to you pinpoint challenges quicker, minimize debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s happening under the hood without needing to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what level. Common logging levels involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information in the course of improvement, INFO for general situations (like thriving start out-ups), WARN for possible issues that don’t break the applying, Mistake for real issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with excessive or irrelevant details. Excessive logging can obscure crucial messages and slow down your process. Give attention to important situations, condition modifications, input/output values, and significant selection details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t probable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. By using a well-imagined-out logging approach, it is possible to lessen the time it will take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To proficiently identify and deal with bugs, builders must method the method just like a detective fixing a thriller. This mentality helps break down intricate difficulties into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Consider the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, gather as much related details as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Up coming, kind hypotheses. Request oneself: What might be creating this behavior? Have any variations not long ago been created for the codebase? Has this situation transpired prior to under similar instances? The target is usually to narrow down possibilities and detect potential culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge inside a managed natural environment. In case you suspect a particular functionality or part, isolate it and confirm if the issue persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay near consideration to little aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-just one mistake, or a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the actual issue, just for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long run troubles and assistance Other people fully grasp your reasoning.
By thinking like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering concealed issues in sophisticated devices.
Generate Tests
Composing assessments is among the simplest ways to enhance your debugging capabilities and overall improvement effectiveness. Exams not simply enable capture bugs early but will also function a security Web that gives you self confidence when building improvements towards your codebase. A well-tested software is much easier to debug mainly because it allows you to pinpoint precisely exactly where and when an issue happens.
Begin with unit exams, which concentrate on personal functions or modules. These little, isolated tests can quickly expose whether a selected bit of logic is working as envisioned. Any time a take a look at fails, you promptly know the place to seem, substantially lowering the time spent debugging. Device assessments are In particular handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assist ensure that many portions of your application work jointly easily. They’re particularly handy for catching bugs that take place in complicated units with a number of parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To check a characteristic thoroughly, you may need to understand its inputs, predicted more info outputs, and edge cases. This standard of comprehending Obviously leads to higher code composition and fewer bugs.
When debugging a concern, creating a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to focus on fixing the bug and enjoy your test pass when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable method—serving to you catch a lot more bugs, speedier plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Resolution after Answer. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable glitches or misreading code you wrote just several hours previously. In this particular condition, your brain becomes significantly less effective at issue-solving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your focus. Many builders report getting the foundation of a difficulty once they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re trapped, a superb rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't a sign of weak spot—it’s a smart method. It presents your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is a lot more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important in the event you take some time to mirror and review what went Incorrect.
Commence by asking by yourself some vital thoughts once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code critiques, or logging? The answers frequently expose blind spots within your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. After some time, you’ll start to see patterns—recurring issues or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've discovered from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a stronger Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your advancement journey. In fact, several of the best builders are not the ones who generate excellent code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So subsequent time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — nevertheless the payoff is large. It makes you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.